TypeError: list indices must be integers or slices, not str
In Python, while working with a list you may come across the error TypeError: list indices must be integers or slices, not str. This error can occur when we try to access elements of a list using String as indexes. In this article, we will understand what this error message means and how to fix it.
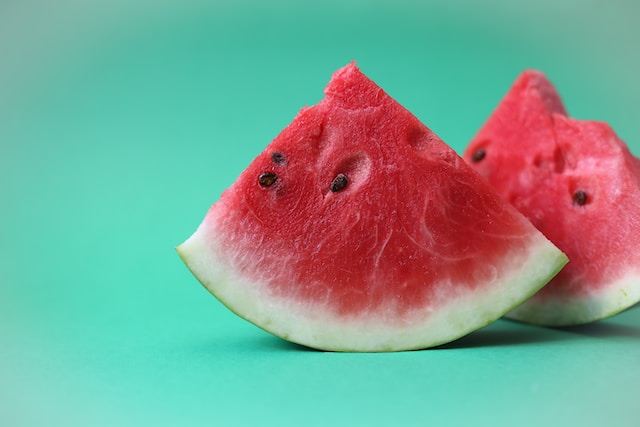
How to Fix the TypeError: list indices must be integers or slices, not str Error in Python
The error message TypeError: list indices must be integers or slices, not str can occur due to a number of reasons some of which are as follows:
Scenario 1: Using String as Indexes
my_list = ["apple", "banana", "cherry", "mango"]
print(my_list["apple"])
Try it yourself -> Online Python editor to check the examples
Output:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: list indices must be integers or slices, not str
Here, we can observe the TypeError: list indices must be integers or slices, not str error is raised because we have tried to access the first element of list my_list using the string “apple” as an index. However, in Python lists are indexed using integers or slices, not strings.
Solution 1: Use Integers
To fix the TypeError: list indices must be integers or slices, not str error, we need to be sure that we are using integers or slices as indices to access elements in a list. Here are a few ways to do this:
my_list = ["apple", "banana", "cherry", "mango"]
print(my_list[0])
Try it yourself -> Online Python editor to check the examples
Output:
apple
The error is resolved now because, in this example, the first element of the list my_list is accessed using the integer index my_list[0].
Note: Indexes in the list start from 0.
Solution 2: Use Slices
To access a range of elements in a list use slice. A slice is a sequence of indices that specifies a range of elements to access. For example:
my_list = [“apple”, “banana”, “cherry”, “mango”]
print(my_list[0:2])
Try it yourself -> Online Python editor to check the examples
Output:
apple, banana
In this example, the first two elements in my_list are accessed using slicing.
Solution 3: Use index() Method
Consider a scenario, where you have a list of student names, and you want to find the index of a specific student in the list. You know the name of the student, but you’re not sure about their index value.
In this case, the index()method can be used to find the index of a specific element in a list. This method returns the index of the first occurrence of the specified element in the list.
students = ["Burhan", "Mikal", "Ayesha", "Aneeza", "Hira", "Usmnan"] student_name = "Aneeza" index = students.index(student_name) print(f"The index of {student_name} in the list is: {index}")
Output:
The index of Aneeza in the list is: 3
In this example, the list of student names called students contained six elements. We wanted to find the index of the student named Aneeza in the list. So, the index() method is used here to do this by passing Aneeza as an argument to the method. The resulting index value was assigned to a variable called index.
Scenario 2: Unconverted Strings
Let’s consider another scenario that can raise this error
colors = ['Red', 'Blue', 'Green', 'Yellow']
choice = input('Enter the index of your preferred color (0, 1, 2 or 3)? ')
print(colors[choice])
Output:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: list indices must be integers or slices, not str
The error TypeError: list indices must be integers or slices, not str is occurred in the given an example because the index of a list should be an integer value or a slice (range of integers), but in the example, the choice variable, which is a string returned by the input() function, is being used as an index to access an element from the colors list.
Solution
colors = ['Red', 'Blue', 'Green', 'Yellow']
choice = int(input('Enter the index of your preferred color (0, 1, 2 or 3)? '))
print(colors[choice])
Output
Enter the index of your preferred color (0, 1, 2 or 3)? 1
Blue
Here, the int() function is used to convert the string to an integer so that it can be used as an index.
Scenario 3: Using a List of Dictionaries
The TypeError: list indices must be integers or slices, not str error can also occur while using lists as dictionaries.
menu_items = [ {'name': 'burger', 'price': 5.99}, {'name': 'fries', 'price': 2.99}, {'name': 'drink', 'price': 1.99} ] order = input("What would you like to order? (burger, fries, drink)") if menu_items['name'] == order: print(f"Your total is ${menu_items['price']}")
Output:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: list indices must be integers or slices, not str
In this example, we have a list of dictionaries representing a menu at a restaurant. We then ask the user to input what they would like to order, and attempt to access the price of that item using the dictionary key name as an index.
The error in this example is caused by trying to access a dictionary key using a list index. Specifically, in the line if menu_items[‘name’] == order:, the menu_items variable is a list of dictionaries, where each dictionary represents a menu item. So, to access a specific menu item, you need to use the index of the dictionary in the list, like menu_items[0][‘name’]. However, in the example code, the code is trying to access the ‘name‘ key of the menu_items list, which is not possible since list indices must be integers or slices, not strings.
Solution:
menu_items = [
{'name': 'burger', 'price': 5.99},
{'name': 'fries', 'price': 2.99},
{'name': 'drink', 'price': 1.99}
]
order = input("What would you like to order? (burger, fries, drink)")
for item in menu_items:
if item['name'] == order:
print(f"Your total is ${item['price']}")
break
else:
print("Sorry, we don't have that item on the menu.")
Output:
What would you like to order? (burger, fries, drink) fries
Your total is $2.99
Conclusion
In conclusion, the TypeError: list indices must be integers or slices, not str error occurs when we try to use a string instead of an integer to index a list, or when we treat a list as a dictionary. To fix this error, use integer indices when accessing list items and make sure that any input values are properly converted to integers as needed. By being mindful of these common pitfalls, we can avoid this error and write more robust Python code.
Good Luck with your Learning !!!