TypeError: string indices must be integers
In python, while working with iterable objects (String, List, Dictionary, and Tuples, etc.) you may encounter this TypeError: string indices must be integers error. In this article, we will understand why this error occurs and what are the possible solutions to fix this error.
“TypeError: string indices must be integers” is a common error that occurs when trying to access a string using a non-integer index. This error can be fixed by using integer indices to access the string and converting non-integer indices to integers if necessary.
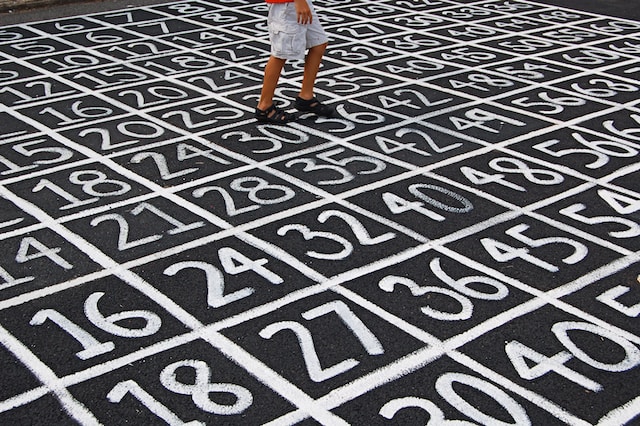
Why does this TypeError: string indices must be integers error occurs?
Before divining into depth, first,z understands what a String is and how Python indexes work. In Python, a String is a sequence of characters, enclosed in single or double quotes. A string has zero-based indexing in Python which means the first character of a string is at index 0, the second character is at index 1 and so on. To understand the reason behind this error let’s consider the below scenarios where you want to access the first element of a string.
Scenario 1: Incorrectly using a string as an index
myString = "Burhan"
first_character = myString['0']
print(first_character)
Output
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: string indices must be integers
You can observe on the execution of the above example, an error TypeError: string indices must be integers occurred. When someone tries to get the index of a string using a non-integer index TypeError: string indices must be integers error will occur. Because in iterable objects the indexes are integers and in the first example we have accidentally used the non-integer string index myString[‘0’].
Scenario 2: Using a floating-point value as an index
myString = “Burhan”
first_character = myString[1.0]
print(first_character)
In this second example, the floating point value 1.0 is used as an index to access the first element of the myString which will cause this error.
Scenario 3: Using a List as an index
myString = "Burhan"
index = [1]
print(myString[index])
Another common cause of this error is using a List as an index. In the third scenario example, the index variable is a list containing a single integer [1]. When we try to access the character at that index in the myString string, the error TypeError: string indices must be integers error will be raised.
Scenario 4: Using a dictionary key as an index
myString = "Burhan"
index = {'pos': 1}
print(myString[index])
In this example, an index variable is a dictionary that contained a key pos with a value of 1. When trying to access the character at that index in the myString string, the error TypeError: string indices must be integers will be raised.
Possible Solutions to Resolve
To resolve this TypeError: string indices must be integers error in all above scenarios use the following solution.
myString = "Burhan" first_character = myString[0] print(first_character)
Try it yourself – Online python editor to check the examples
Output
B
In this example, a string myString contained the name Burhan. To get the first index of a string we have used an integer index 0 which is enclosed inside square brackets myString[0]. On execution of the above code, an error is removed and B is returned which is the first character of myString.
Scenario 5: Using Slice Notation in String
myString = "Hello! How are you?"
myString[0,5]
Output:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: string indices must be integers
In this example, the string myString contained the value “Hello! How are you?“. And the myString[0,5] is used to extract a slice of the string starting from index 0 to ending at index 5. However, the syntax 0,5 is not valid for slice notation in Python.
Possible Solutions to Resolve
myString = "Hello! How are you?" mySubstring = myString[0:5] print(mySubstring)
Try it yourself – Online python editor to check the examples
Output
Hello
In Python, we can use slice notation to extract a portion of a string. The slice notation consists of two indices separated by a colon : where the first index indicates the starting position of the slice (inclusive) and the second index indicates the ending position of the slice (exclusive).
We can observe, in the above code snippet, colon 0:5 is used to separate the starting and ending indices as of string Instead of a comma 0,5 which correctly extracted the slice Hello of the string mySubstring from the index 0 to index 4(inclusive).
Conclusion:
In conclusion, the TypeError: string indices must be integers error is a common error that can occur when working with strings in Python. It typically arises when a non-integer value is used as an index to access the elements of a string. However, this error can be fixed by making sure that indices are integers. We have also seen a scenario where string slice notation is used incorrectly which is one of the reasons behind this error. By following the guidelines provided in this article you will hopefully resolve this error.
Good Luck with your Learning !!